Creating applications for commonly used python code can be useful because it allows non-coders to be able to use the code without coding knowledge and can simplify the execution of the code by using a graphical user interface (GUI). Visikol has experience in creating applications for customers to execute machine learning models or other types of analyses and for internal use to simplify time consuming tasks. In this post, I will explain how you can create your own application in python through an example script for a simple image viewing application. However, before we get started, the packages you will need are tkinter, PyInstaller and Pillow. The IDE I will be using is PyCharm.
STEP 1: Setting up the GUI
In order to make the code easy to use, we must first write the script that will do all the heavy lifting in the background. For this, we shall use tkinter to set up the GUI for the application and program the widgets in the GUI to perform the operations for viewing images. The application will allow users to select an image file from their computer and view it in the software, so we will need to code a way for users to select the file and a way for users to then view the selected image. To start with, we will need to import the proper packages and initialize the window for the GUI as seen in Figure 1 where the “window” variable will be the canvas that we can then place our widgets into.
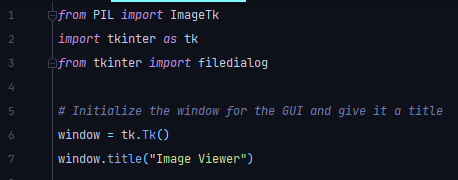
Figure 1. Initialization of the packages to be used in the script along with the window for holding the widgets for the GUI.
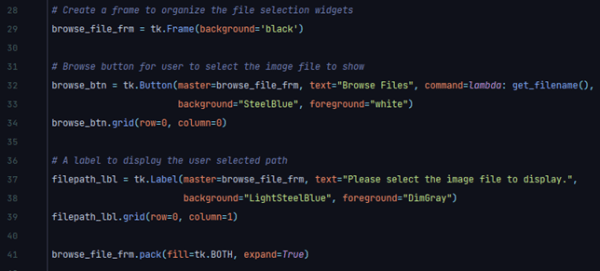
Figure 2. Code to set up the browse files button and file path label for user selection of the image file to be viewed.
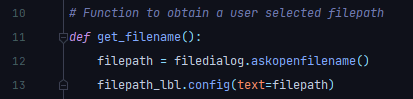
Figure 3. The function that gets called when pressing the browse files button to prompt the user to select the image file of choice and update the file path label.
STEP 2: Image File User Input
After setting up the window for the GUI, we will set up the widgets that the user will see and add functionality to them. First, we will set up the widgets for selecting the image file to open as seem in Figure 2. To accomplish this, we will create a button to allow users to browse the file explorer for their image and a label to display the path they have chosen. Additionally, these widgets will be placed inside a frame widget to keep the widgets organized and allow for easy styling. To set these up, we will use the Frame, Button, and Label functions within tkinter, which shall set up the widget with arguments for the styling and adding functionality being passed to them. To place these widgets onto the window, we shall use the pack and grid functions on the widget objects which handle the placement of the widgets in the window.
When creating the button, we will have to define a function to pass to the command argument that will perform the task of prompting the user for the image they wish to select and update the label to display the chosen path. This shall be done through a defined function called get_filename, which will use askopenfilename to prompt the user to select the image file from the file explorer and store the path of the image into a variable, which will then be used to update the label to show the selected image path as seen in Figure 3.
STEP 3: Displaying the Image
Once the file selection functionality is set up, we will set up the button used to view the selected image. This can be seen in Figure 4 where similar steps for button creation as previously shown can be seen and the button is put inside a frame for ease of styling. Additionally, the function attached to the button can be seen in Figure 5 where the image path is obtained from the filepath_lbl widget from before and the path is used to load in the image. Once the image is loaded, we will then display it within a label widget and then add that widget to the same frame as the run button. After this is complete, the GUI and its functionality are now fully coded and when running the script, our GUI will look like it does in Figure 6.
STEP 4: Creating the Application
Now that the script is fully functional, it would be okay to leave it in the form that it is in, however, we would like to package it into an application to allow others to easily execute the script without knowledge about coding. To do this, we will need to go to the terminal in PyCharm and point to the directory that holds the script by typing “cd {directory path}”. Once the terminal points to the correct directory, we shall then use PyInstaller to package the application by typing in “PyInstaller –onefile {script name}”. After the code has been executed in the terminal, PyInstaller will then process the script into an application and the supporting files will then be placed within the directory of the script. The executable will be in the dist folder, and you can then distribute that file to anyone to be able to run your code without coding knowledge.
If you are interested in developing biomedical algorithms to be packaged into software for use in image analysis or machine learning tasks, please contact Visikol as they have experience in developing applications as executables or software that runs off AWS cloud servers.
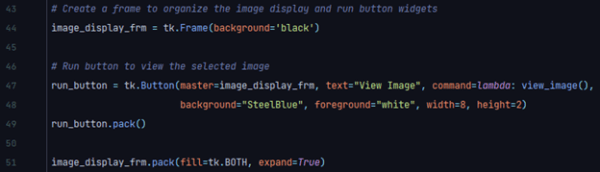
Figure 4. Code to set up the view image button.
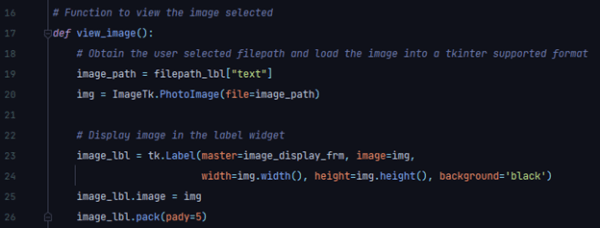
Figure 5. The function called when pressing the view image button that loads in the image and displays it in the GUI window using a label widget.
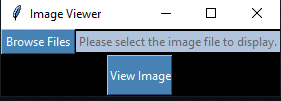
Figure 6. The window for the GUI that shows all the widgets that were coded in for selecting the file and viewing the image.